What does the following code do, assuming that dblValue1 and dblValue2 are both declared as doubles?
try
{
dblValue1 = Double.Parse( txtInput1.Text );
dblValue2 = Double.Parse( txtInput2.Text );
txtOutput.Text = Convert.ToString( dblValue1 * dblValue2 );
}
catch ( FormatException formatExceptionParameter )
{
MessageBox.Show(
"Please enter decimal values.",
"Invalid Number Format",
MessageBoxButtons.OK, MessageBoxIcon.Error );
}
This code multiplies two doubles if both inputs in txtInput1 and txtInput2 can be converted to type double (that is, they are decimal or integer values). Otherwise it displays an error message dialog that informs the user to enter decimal values in the TextBoxes. The complete code reads:
// Multiplier.cs
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Multiplier
{
///
/// Summary description for FrmMultiplier.
///
public class FrmMultiplier : System.Windows.Forms.Form
{
// Label and TextBox for first value
private System.Windows.Forms.Label lblValue1;
private System.Windows.Forms.TextBox txtInput1;
// Label and TextBox for second value
private System.Windows.Forms.Label lblValue2;
private System.Windows.Forms.TextBox txtInput2;
// Label and TextBox for output
private System.Windows.Forms.Label lblProduct;
private System.Windows.Forms.TextBox txtOutput;
// Button to perform multiplication
private System.Windows.Forms.Button btnMultiply;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmMultiplier()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmMultiplier() );
}
// handles btnMultiply's Click event
private void btnMultiply_Click(
object sender, System.EventArgs e )
{
double dblValue1;
double dblValue2;
try
{
dblValue1 = Double.Parse( txtInput1.Text );
dblValue2 = Double.Parse( txtInput2.Text );
txtOutput.Text =
Convert.ToString( dblValue1 * dblValue2 );
}
catch ( FormatException formatExceptionParameter )
{
MessageBox.Show(
"Please enter decimal values.",
"Invalid Number Format",
MessageBoxButtons.OK, MessageBoxIcon.Error );
}
} // end method btnMultiply_Click
} // end class FrmMultiply
}
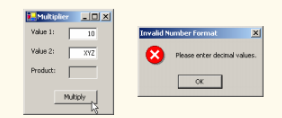
You might also like to view...
When invoking a function, the data type and position of each argument does not need to agree with the data type and position of its corresponding parameter.
Answer the following statement true (T) or false (F)
What is the purpose of the wildcard bits?
What will be an ideal response?
Define viewport:
a. Makes min-width and max-width media queries work in older versions of IE. b. Each width at which content would benefit from adjustment. c. Free, browser-based tool that provides views that map to a few popular device sizes. d. Area within browser that displays page, both on desktop and mobile browsers.
Web applications have access to device capabilities, like the accelerometer or location services.
Answer the following statement true (T) or false (F)