Find the error(s) in the following code. This method should modify the Age field of strUserName.
objUpdateAge[ "Age" ].Value = intAge;
objUpdateAge.Parameters[ "Original_NAME" ].Value =
strUserName;
objUpdateAge.ExecuteNonQuery();
objOleDbConnection.Close();
objUpdateAge[ "Age" ].Value = intAge;
The UPDATE statement on line 1 is malformed. Also, no connection is made to the database before the UPDATE is attempted. For this example, a sample database named Sample.mdb has been provided in the bin\debug directory. Follow the instructions previously presented in this tutorial to establish a connection to the database. The complete incorrect code reads:
// UpdateAge.cs (Incorrect)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Data.OleDb;
namespace UpdateAge
{
///
/// Summary description for FrmUpdateAge.
///
public class FrmUpdateAge : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblAgeOut;
private System.Windows.Forms.Label lblNameOut;
private System.Windows.Forms.Label lblName;
private System.Windows.Forms.Label Label1;
private System.Data.OleDb.OleDbConnection objOleDbConnection;
private System.Data.OleDb.OleDbCommand objUpdateAge;
private System.Data.OleDb.OleDbCommand objSelectAge;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmUpdateAge()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmUpdateAge() );
}
// updates an age in the database "sample.mdb" located in
// the "bin\debug" directory
private void FrmUpdateAge_Load(
object sender, System.EventArgs e )
{
int intAge = 27;
string strUserName = "Bill";
objUpdateAge[ "Age" ].Value = intAge;
objUpdateAge.Parameters[ "Original_NAME" ].Value =
strUserName;
objUpdateAge.ExecuteNonQuery();
objOleDbConnection.Close();
// display the updated data
objOleDbConnection.Open();
OleDbDataReader objReader =
objSelectAge.ExecuteReader();
objReader.Read();
lblAgeOut.Text = Convert.ToString( objReader[ "Age" ] );
objReader.Close();
objOleDbConnection.Close();
} // end method FrmUpdateAge_Load
} // end class FrmUpdateAge
}
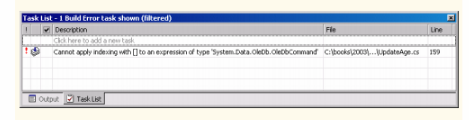
The complete corrected code should read:
// UpdateAge.cs (Correct)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Data.OleDb;
namespace UpdateAge
{
///
/// Summary description for FrmUpdateAge.
///
public class FrmUpdateAge : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblAgeOut;
private System.Windows.Forms.Label lblNameOut;
private System.Windows.Forms.Label lblName;
private System.Windows.Forms.Label Label1;
private System.Data.OleDb.OleDbConnection objOleDbConnection;
private System.Data.OleDb.OleDbCommand objUpdateAge;
private System.Data.OleDb.OleDbCommand objSelectAge;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmUpdateAge()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmUpdateAge() );
}
// updates an age in the database "sample.mdb" located in
// the "bin\debug" directory
private void FrmUpdateAge_Load(
object sender, System.EventArgs e )
{
int intAge = 27;
string strUserName = "Bill";
objUpdateAge.Parameters[ "Age" ].Value = intAge;
objUpdateAge.Parameters[ "Original_NAME" ].Value =
strUserName;
objOleDbConnection.Open();
objUpdateAge.ExecuteNonQuery();
objOleDbConnection.Close();
// display the updated data
objOleDbConnection.Open();
OleDbDataReader objReader =
objSelectAge.ExecuteReader();
objReader.Read();
lblAgeOut.Text =
Convert.ToString( objReader[ "Age" ] );
objReader.Close();
objOleDbConnection.Close();
} // end method FrmUpdateAge_Load
} // end class FrmUpdateAge
}
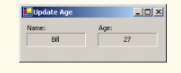
You might also like to view...
When a process divides itself into two or more tasks running simultaneously, each task is referred to as a:
A) Strand B) Semi-task C) Mini program D) Thread
Bob sends Alice an encrypted message using RSA. What key would Alice need to use to decrypt the message?
A. Public key B. Private key C. Shared key D. The same key that Bob used to encrypt the message
?In terms of e-commerce infrastructure, the Web server's required amount of storage capacity and computing power depends primarily on two things: the software that must run on the server and the quantity of content on the e-commerce site.
Answer the following statement true (T) or false (F)
What device connects a LAN?s switch to the next network?
A. Router B. Transceiver C. MAUS D. Gateway