What is returned by the following code? Assume that GetStockPrices is a method that returns a 2-by-31 array, with the first row containing the stock price at the beginning of the day and the last row containing the stock price at the end of the day, for each day of the month.
int[] Mystery()
{
int[,] intPrices = new int[ 2, 31 ];
intPrices = GetStockPrices();
int[] intResult = new int[ 31 ];
for ( int intI = 0; intI < intResult.Length; intI++ )
{
intResult[ intI ] = intPrices[ 1, intI ] -
intPrices[ 0, intI ];
}
return intResult;
} // end method Mystery
The method returns a one-dimensional array containing the daily stock price
change for each day of the month. The complete code reads:
// StockPrices.cs
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace StockPrices
{
///
/// Summary description for FrmStockPrices.
///
public class FrmStockPrices : System.Windows.Forms.Form
{
private System.Windows.Forms.ListBox lstPriceChange;
private System.Windows.Forms.Label lblChange;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmStockPrices()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmStockPrices() );
}
// displays the daily change in stock price over 1 month
private void FrmStockPrices_Load(
object sender, System.EventArgs e )
{
int[] intPrices;
int intLength;
intPrices = Mystery();
intLength = intPrices.Length;
// add a header to indicate the columns meaning
lstPriceChange.Items.Add( "Day\tChange" );
// print the array with proper formatting
for ( int intI = 0; intI < intLength; intI++ )
{
lstPriceChange.Items.Add( intI + "\t" +
Convert.ToString( intPrices[ intI ] ).PadLeft( 10,
Convert.ToChar( " " ) ) );
}
} // end method FrmStockPrices_Load
// calculates the daily change in stock price for one month
int[] Mystery()
{
int[,] intPrices = new int[ 2, 31 ];
intPrices = GetStockPrices();
int[] intResult = new int[ 31 ];
for ( int intI = 0; intI < intResult.Length; intI++ )
{
intResult[ intI ] = intPrices[ 1, intI ] -
intPrices[ 0, intI ];
}
return intResult;
} // end method Mystery
// returns a 2D array of stock prices
int[,] GetStockPrices()
{
int[,] intStocks = {
{ 13, 18, 17, 15, 10, 9, 10, 12, 11, 13, 15, 20, 23,
25, 20, 18, 19, 20, 14, 12, 10, 9, 10, 12, 13, 14,
15, 16, 13, 14, 20 },
{ 18, 17, 15, 10, 9, 10, 12, 11, 13, 15, 20, 23, 25,
20, 18, 19, 20, 14, 12, 10, 9, 10, 12, 13, 14, 15,
16, 13, 14, 20, 22 }
};
return intStocks;
} // end method GetStockPrices
} // end class FrmStockPrices
}
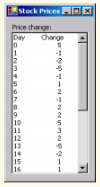
Computer Science & Information Technology
You might also like to view...
The actual value that you pass to an object via a message is called a parameter.
Answer the following statement true (T) or false (F)
Computer Science & Information Technology
Which of the following frames can be thought of as the hotspot for a button?
A. Over B. Up C. Hit D. Down
Computer Science & Information Technology
Manipulating data in ________ is preferable to changing data in Access
Fill in the blank(s) with correct word
Computer Science & Information Technology
You can add apps or Windows ____________________ to the Start menu or customize the way the Start menu looks and functions.
Fill in the blank(s) with the appropriate word(s).
Computer Science & Information Technology