What does this code do? What is the result of the following code?
string strPath1 = "oldfile.txt";
string strPath2 = "newfile.txt";
string strLine;
StreamWriter objStreamWriter;
objStreamWriter = new StreamWriter( strPath2 );
StreamReader objStreamReader;
objStreamReader = new StreamReader( strPath1 );
while ( objStreamReader.Peek() > -1 )
{
strLine = objStreamReader.ReadLine();
objStreamWriter.WriteLine( strLine );
}
objStreamWriter.Close();
objStreamReader.Close();
The code copies the contents of oldfile.txt to newfile.txt. After the file trans-
fer is completed, the files are closed by closing the StreamReader and StreamWriter. Note that if a blank line is encountered, copying stops at the blank line. The complete code reads:
// CopyFile.cs
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.IO;
namespace CopyFile
{
///
/// Summary description for FrmCopyFile.
///
public class FrmCopyFile : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblCopy;
private System.Windows.Forms.Label lblOriginal;
private System.Windows.Forms.TextBox txtCopy;
private System.Windows.Forms.TextBox txtOriginal;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmCopyFile()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmCopyFile() );
}
// copies newfile.txt to oldfile.txt and displays both
private void FrmCopyFile_Load(
object sender, System.EventArgs e )
{
string strPath1 = "oldfile.txt";
string strPath2 = "newfile.txt";
string strLine;
StreamWriter objStreamWriter;
objStreamWriter = new StreamWriter( strPath2 );
StreamReader objStreamReader;
objStreamReader = new StreamReader( strPath1 );
while ( objStreamReader.Peek() > -1 )
{
strLine = objStreamReader.ReadLine();
objStreamWriter.WriteLine( strLine );
}
objStreamWriter.Close();
objStreamReader.Close();
// display both files in TextBoxes
objStreamReader = new StreamReader( strPath1 );
while ( objStreamReader.Peek() > -1 )
{
strLine = objStreamReader.ReadLine();
txtCopy.Text += strLine; + "\r\n";
txtOriginal.Text += strLine + "\r\n";
}
objStreamWriter.Close();
objStreamReader.Close();
} // end method FrmCopyFile_Load
} // end class FrmCopyFile
}
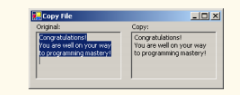
You might also like to view...
A___________ is a group of related files.
a) field b) database c) collection d) byte
Options for changing row height and column width are in the Table Tools contextual Design tab
Indicate whether the statement is true or false
The Normal style defines line spacing to single and does not insert any additional blank space between lines when you press the ENTER key.
Answer the following statement true (T) or false (F)
Style sheets that are part of the head content of an individual web page are referred to as _____________ style sheets.
Fill in the blank(s) with the appropriate word(s).