Find the error(s) in the following code. The following method should create a new Shape object with intNumberSides sides. Assume the Shape class
private void ManipulateShape( int intNumberSides )
{
Shape objShape = new Shape( 3 );
Shape.m_intSides = intNumberSides;
} // end method ManipulateShape
The method should create a Shape object with intNumberSides sides—not a
Shape object with 3 sides. Also, a private variable (m_intSides) cannot be accessed from outside the class. The complete incorrect code reads:
// Manipulate.cs (Incorrect)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using Shapes;
namespace Manipulate
{
///
/// Summary description for FrmManipulate.
///
public class FrmManipulate : System.Windows.Forms.Form
{
private System.Windows.Forms.Button btnChange;
private System.Windows.Forms.TextBox txtInput;
private System.Windows.Forms.Label lblChangeTo;
private System.Windows.Forms.Label lblSidesOut;
private System.Windows.Forms.Label lblSides;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmManipulate()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmManipulate() );
}
// updates the number of sides in the shape
private void btnChange_Click(
object sender, System.EventArgs e )
{
int intNewSides;
Shape objNewShape;
intNewSides = Int32.Parse( txtInput.Text );
objNewShape = ManipulateShape( intNewSides );
lblSidesOut.Text = Convert.ToString( objNewShape.Side );
} // end method btnChange_Click
// creates a new shape object with intNumberSides sides
private void ManipulateShape( int intNumberSides )
Shape objShape = new Shape( 3 );
m_intSides is declared private Shape.m_intSides = intNumberSides;
} // end method ManipulateShape
} // end class FrmManipulate
}
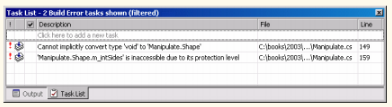
Computer Science & Information Technology
You might also like to view...
__________ can be used to develop a variety of elliptic curve cryptography schemes.
A. Elliptic curve arithmetic B. Binary curve C. Prime curve D. Cubic equation
Computer Science & Information Technology
What is an I S quality circle?
What will be an ideal response?
Computer Science & Information Technology
In a form or report, a ________ arranges records by a common value and allows the addition of summary data for each arrangement
A) group B) set C) cluster D) collection
Computer Science & Information Technology
A recipient list is also known as a(n) ________
Fill in the blank(s) with correct word
Computer Science & Information Technology