Find the error(s) in the following code. This is the definition for a Click event handler for a Button. This event handler should draw a filled rectangle on a PictureBox control.
private void btnDrawImage_Click(
object sender, System.EventArgs e)
{
// create an orange colored brush
SolidBrush objBrush = new SolidBrush( Orange );
// create a Graphics object to draw on the PictureBox
Graphics objGraphics = picPictureBox.AcquireGraphics();
// draw a filled rectangle
objGraphics.FillRectangle( objBrush, 2, 3, 40, 30 );
} // end method btnDrawImage_Click
When specifying a color for the SolidBrush you must precede the color name with
“Color.” You cannot just write the color name. Also, to retrieve the Graphics object, the CreateGraphics method must be used, not AcquireGraphics. The complete incorrect code reads:
// DrawRectangle.cs (Incorrect)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace DrawRectangle
{
///
/// Summary description for FrmDrawRectangle.
///
public class FrmDrawRectangle : System.Windows.Forms.Form
{
private System.Windows.Forms.Button btnDrawImage;
private System.Windows.Forms.Label lblImage;
private System.Windows.Forms.PictureBox picPictureBox;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmDrawRectangle()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmDrawRectangle() );
}
// draws a rectangle in the picture box
private void btnDrawImage_Click(
object sender, System.EventArgs e )
{
// create an orange colored brush
SolidBrush objBrush = new SolidBrush( Orange );
// create a Graphics object to draw on the PictureBox
Graphics objGraphics = picPictureBox.AcquireGraphics();
// draw a filled rectangle
objGraphics.FillRectangle( objBrush, 2, 3, 40, 30 );
} // end method btnDrawImage_Click
} // end class FrmDrawRectangle
}
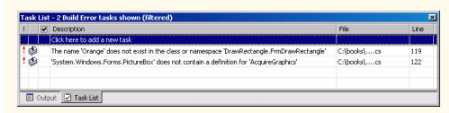
The complete corrected code should read:
// DrawRectangle.cs (Correct)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace DrawRectangle
{
///
/// Summary description for FrmDrawRectangle.
///
public class FrmDrawRectangle : System.Windows.Forms.Form
{
private System.Windows.Forms.Button btnDrawImage;
private System.Windows.Forms.Label lblImage;
private System.Windows.Forms.PictureBox picPictureBox;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmDrawRectangle()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmDrawRectangle() );
}
// draws a rectangle in the picture box
private void btnDrawImage_Click(
object sender, System.EventArgs e )
{
// create an orange colored brush
SolidBrush objBrush = new SolidBrush( Color.Orange );
// create a Graphics object to draw on the PictureBox
Graphics objGraphics = picPictureBox.CreateGraphics();
// draw a filled rectangle
objGraphics.FillRectangle( objBrush, 2, 3, 40, 30 );
} // end method btnDrawImage_Click
} // end class FrmDrawRectangle
}
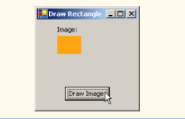
You might also like to view...
_________ is the process of combining two or more values to create a single value
Fill in the blank(s) with correct word
What are the benefits of normalization?
What will be an ideal response?
What is the protocol developed by Cisco for enterprise-wide routing environments?
A. RIP B. OSPF C. BGP D. EIGRP
With automatic ____, the Java programmer is freed of the complex task of deciding when and where to safely release memory.
A. stack access B. memory access C. garbage collection D. heap management