What does the following code do?
1 int m_intAge;
2 objSelectAgeData.Parameters[ "Name" ].Value = "Bob";
3
4 objOleDbConnection.Open();
5
6 OleDbDataReader objReader =
7 objSelectAgeData.ExecuteReader();
8
9 objReader.Read();
10
11 m_intAge = Convert.ToInt32( objReader[ "Age" ] );
12
13 objReader.Close();
14 objOleDbConnection.Close();
This code segment connects to a database and retrieves the Age data for the person with the name Bob. This data is then stored in m_intAge. The code then closes both the data reader and the connection to the database. For this example, a sample database named Employees.mdb has been provided in the bin\debug directory. Follow the instructions previously presented in this tutorial to establish a connection to the database. The complete code reads:
// GetAge.cs
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Data.OleDb;
namespace GetAge
{
///
/// Summary description for FrmGetAge.
///
public class FrmGetAge : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblAgeOut;
private System.Windows.Forms.Label lblAge;
private System.Windows.Forms.Label lblNameOut;
private System.Windows.Forms.Label lblName;
private System.Data.OleDb.OleDbConnection objOleDbConnection;
private System.Data.OleDb.OleDbCommand objSelectAgeData;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmGetAge()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run(new FrmGetAge());
}
// retrieves and displays the age of Bob from "Employees.mdb"
// in the "bin\debug" directory
private void FrmGetAge_Load(
object sender, System.EventArgs e )
{
int m_intAge;
objSelectAgeData.Parameters[ "Name" ].Value = "Bob";
objOleDbConnection.Open();
OleDbDataReader objReader =
objSelectAgeData.ExecuteReader();
objReader.Read();
m_intAge = Convert.ToInt32( objReader[ "Age" ] );
objReader.Close();
objOleDbConnection.Close();
lblAgeOut.Text = Convert.ToString( m_intAge );
} // end method FrmGetAge_Load
} // end class FrmGetAge
}
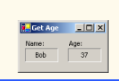
You might also like to view...
Discuss the functionality required of a replication server.
What will be an ideal response?
____ means that you can place one repetition structure entirely within another repetition structure.
A. Encasing B. Subordinating C. Nesting D. Folding
Match the following Word features with their association:
I. Bibliography A. topics and page numbers in a document II. Table of authorities B. considered by many as a Works Cited III. Table of figures C. a reference of captions IV. Table of contents D. used in legal documents V. Index E. headings as they appear in a document
A docstring is a string enclosed in double quotes that will be displayed when Python's help function is run on a resource.
Answer the following statement true (T) or false (F)