The code that follows uses a for loop to sum the elements in an array. Find the error(s) in the following code:
int SumArray()
{
int intSum = 0;
int[] intNumbers = { 1, 2, 3, 4, 5, 6, 7, 8 };
for ( int intCounter = 0; intCounter <= intNumbers.Length;
intCounter++ )
{
intSum += intNumbers[ intCounter ];
}
return intSum;
} // end method SumArray
Array intNumbers does have intNumbers.Length number of elements, but the
indices are zero through intNumbers.Length – 1. The for loop increments intCounter beyond the highest index in the array which results in a run-time error. To correct the error, change the loop-continuation condition to use < rather than <=. The complete incorrect code reads:
// SumArray.cs (Incorrect)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace SumArray
{
///
/// Summary description for FrmSumArray.
///
public class FrmSumArray : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblSumOutput;
private System.Windows.Forms.Label lblSum;
private System.Windows.Forms.Label lblArrayContents;
private System.Windows.Forms.Label lblArray;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmSumArray()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmSumArray() );
}
// prints the result of the SumArray method
private void FrmSumArray_Load(
object sender, System.EventArgs e )
{
lblSumOutput.Text = Convert.ToString( SumArray() );
} // end method FrmSumArray_Load
// returns the sum of all of an array's elements
int SumArray()
{
int intSum = 0;
int[] intNumbers = { 1, 2, 3, 4, 5, 6, 7, 8 };
for ( int intCounter = 0; ;
intCounter++ )
{
intSum += intNumbers[ intCounter ];
}
return intSum;
} // end method SumArray
} // end class FrmSumArray
}
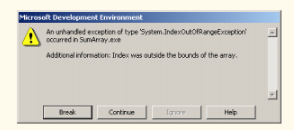
The complete corrected code should read:
// SumArray.cs (Correct)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace SumArray
{
///
/// Summary description for FrmSumArray.
///
public class FrmSumArray : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblSumOutput;
private System.Windows.Forms.Label lblSum;
private System.Windows.Forms.Label lblArrayContents;
private System.Windows.Forms.Label lblArray;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmSumArray()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmSumArray() );
}
// prints the result of the SumArray method
private void FrmSumArray_Load(
object sender, System.EventArgs e )
{
lblSumOutput.Text = Convert.ToString( SumArray() );
} // end method FrmSumArray_Load
// returns the sum of all of an array's elements
int SumArray()
{
int intSum = 0;
int[] intNumbers = { 1, 2, 3, 4, 5, 6, 7, 8 } ;
for ( int intCounter = 0; intCounter < intNumbers.Length;
intCounter++ )
{
intSum += intNumbers[ intCounter ];
}
return intSum;
} // end method SumArray
} // end class FrmSumArray
}
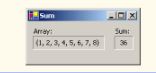
You might also like to view...
_________________________ allow additional functionality to be implemented in an IPv6 packet.
Fill in the blank(s) with the appropriate word(s).
A class is a definition or template used to define the properties and methods that represents objects.
Answer the following statement true (T) or false (F)
Explain how the Clipboard is used when relocating text.
What will be an ideal response?
_____________ involves comparing the live sample to the reference sample in the database.
Fill in the blank(s) with the appropriate word(s).