Assume that intNumbers is an int array. What does intTempArray contain after the execution of the for statement?
int intLength = intNumbers.Length;
int[] intTempArray = new int[ intLength ];
intLength--;
for ( int intI = intLength; intI >= 0; intI-- )
{
intTempArray[ intI ] = intNumbers[ intLength - intI ];
}
This code takes the contents of the parameter intNumbers and reverses the order
of its contents, which are then stored in intTempArray. The complete code reads:
// ReverseArray.cs
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace ReverseArray
{
///
/// Summary description for FrmReverse.
///
public class FrmReverse : System.Windows.Forms.Form
{
private System.Windows.Forms.ListBox lstReversed;
private System.Windows.Forms.Label lblReversed;
private System.Windows.Forms.ListBox lstOriginal;
private System.Windows.Forms.Label lblOriginal;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmReverse()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmReverse() );
}
// calls the Mystery method
private void FrmReverse_Load(
object sender, System.EventArgs e )
{
int[] intNumbers = { 1, 2, 3, 4, 5 };
int intLength = intNumbers.Length;
int[] intTempArray = new int[ intLength ];
intLength--;
for ( int intI = intLength; intI >= 0; intI-- )
{
intTempArray[ intI ] = intNumbers[ intLength - intI ];
}
// instructs the ListBox to fill itself with this array
lstOriginal.DataSource = intNumbers;
// calls Mystery, then instructs the ListBox to fill itself
// with the new array
lstReversed.DataSource = intTempArray;
} // end method FrmReverse_Load
} // end class FrmReverse
}
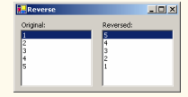
Computer Science & Information Technology
You might also like to view...
Which of the following is a storage class?
A. global B. local C. automatic D. Both A and B are storage classes.
Computer Science & Information Technology
FIGURE AC 5-1
Referring to the figure above, in this report, ____ appear in controls.
A. the Teacher ID label B. the records shown below the Teacher ID label C. both a. and b. D. neither a. nor b.
Computer Science & Information Technology
Describe the discretionary access control (DAC) model.
What will be an ideal response?
Computer Science & Information Technology
Which runlevel represents multiuser mode without network server services?
A. 0 B. 1 C. 2 D. 5
Computer Science & Information Technology