Find the error(s) in the following code (the method should assign the value 14 to vari- able intResult).
int Sum()
{
string strNumber = "4";
int intNumber = 10;
int intResult;
intResult = strNumber + intNumber;
return intResult;
} // end method Sum
The code must explicitly convert strNumber to int. The complete incorrect code
reads:
// Sum.cs (Incorrect)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Sum
{
///
/// Summary description for FrmSum.
///
public class FrmSum : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblSumOut;
private System.Windows.Forms.Label lblNumber2Out;
private System.Windows.Forms.Label lblNumber1Out;
private System.Windows.Forms.Label lblSum;
private System.Windows.Forms.Label lblNumber2;
private System.Windows.Forms.Label lblNumber1;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmSum()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmSum() );
}
// prints the result of the Sum method
private void FrmSum_Load( object sender, System.EventArgs e )
{
lblSumOut.Text = Convert.ToString( Sum() );
} // end method FrmSum_Load
// takes the sum of a string and an int
int Sum()
{
string strNumber = "4";
int intNumber = 10;
int intResult;
intResult = strNumber + intNumber;
return intResult;
} // end method Sum
} // end class FrmSum
}
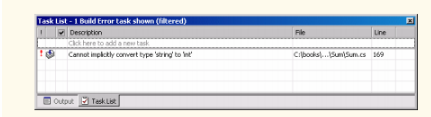
The complete corrected code should read:
// Sum.cs (Correct)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Sum
{
///
/// Summary description for FrmSum.
///
public class FrmSum : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblSumOut;
private System.Windows.Forms.Label lblNumber2Out;
private System.Windows.Forms.Label lblNumber1Out;
private System.Windows.Forms.Label lblSum;
private System.Windows.Forms.Label lblNumber2;
private System.Windows.Forms.Label lblNumber1;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmSum()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmSum() );
}
// prints the result of the Sum method
private void FrmSum_Load( object sender, System.EventArgs e )
{
lblSumOut.Text = Convert.ToString( Sum() );
} // end method FrmSum_Load
// takes the sum of a string and an integer
int Sum()
{
string strNumber = "4";
int intNumber = 10;
int intResult;
intResult = Int32.Parse( strNumber ) + intNumber;
return intResult;
} // end method Sum
} // end class FrmSum
}
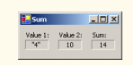
Computer Science & Information Technology
You might also like to view...
Identify the letter of the protection choice that best matches the button or definition.
A. Encrypt with Password B. Mark as Final C. Restrict Permission by People D. Protect Workbook Structure E. Protect Current Sheet
Computer Science & Information Technology
Explain why it is sometimes beneficial to merge cells in a table, as well as when it might be best to split cells.
What will be an ideal response?
Computer Science & Information Technology
?A label accepts text, such as names, dates, and passwords, and is often called an input field.
Answer the following statement true (T) or false (F)
Computer Science & Information Technology
What is the index number of the last element of an array with 29 elements? Group of answer choices
A. 29 B. 28 C. 0 D. Programmer-defined
Computer Science & Information Technology