What does this code do? What is assigned to strResult when the following code executes?
string strWord1 = "CHORUS";
string strWord2 = "d i n o s a u r";
string strWord3 = "The theme is string.";
string strResult;
strResult = strWord1.ToLower();
strResult = strResult.Substring( 4 );
strWord2 = strWord2.Replace( " ", "" );
strWord2 = strWord2.Substring( 4, 4 );
strResult = strWord2 + strResult;
strWord3 = strWord3.Substring(
strWord3.IndexOf( " " ) + 1, 3 );
strResult = strWord3.Insert( 3, strResult );
After assigning initial values to strings strWord1, strWord2 and strWord3, the
code above changes the word "CHORUS" to all lowercase letters, resulting in "chorus" being assigned to strResult. strResult then is assigned the substring of "chorus" beginning at the fifth character (index 4), "u", resulting in strResult’s value as "us". The next line of code deletes spaces from strWord2, resulting in the word "dinosaur". The substring of "dinosaur" beginning at the fifth character and of length 4 ("saur") is then assigned to strWord2. Then, the value of strResult ("us") is appended to the end of strWord2 ("us") and placed in strResult, yielding "saurus". Following that, strWord3 is assigned a sub-string of itself beginning one character after the first space character and of length 3 ("the").
Finally, the value "saurus" (strResult) is inserted into strWord3 at the location of the fourth character (the end of the string) and assigned to strResult. The final value of strResult is the word "thesaurus". The complete code reads:
// StringTest.cs
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace StringTest
{
///
/// Summary description for FrmStringTest.
///
public class FrmStringTest : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblResultOut;
private System.Windows.Forms.Label lblString3Out;
private System.Windows.Forms.Label lblString2Out;
private System.Windows.Forms.Label lblString1Out;
private System.Windows.Forms.Label lblResult;
private System.Windows.Forms.Label lblString3;
private System.Windows.Forms.Label lblString2;
private System.Windows.Forms.Label lblString1;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmStringTest()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmStringTest() );
}
// manipulates several strings and displays the result
private void FrmStringTest_Load(
object sender, System.EventArgs e )
{
string strWord1 = "CHORUS";
string strWord2 = "d i n o s a u r";
string strWord3 = "The theme is string.";
string strResult;
strResult = strWord1.ToLower();
strResult = strResult.Substring( 4 );
strWord2 = strWord2.Replace( " ", "" );
strWord2 = strWord2.Substring( 4, 4 );
strResult = strWord2 + strResult;
strWord3 = strWord3.Substring(
strWord3.IndexOf( " " ) + 1, 3 );
strResult = strWord3.Insert( 3, strResult );
lblResultOut.Text = strResult;
} // end method FrmStringTest_Load
} // end class FrmStringTest
}
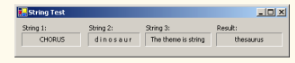
You might also like to view...
What issue could happen if one wireless client is running 802.11b and another is running 802.11g, and both connect to the wireless router at the same time?
a. There are no issues. b. The wireless router will select 802.11g for setting the data transfer rate. c. The wireless router will select 802.11b for setting the data transfer rate. d. The access point will temporarily shut down until one client goes offline.
Be interested enough in what you are saying that you do not use a(n) ________ voice to deliver the message
Fill in the blank(s) with correct word
Establishing performance measures and creating project way points simplifies project monitoring. __________
Answer the following statement true (T) or false (F)
Depending on your settings, a History list might contain icons for websites visited several weeks ago, last week, and every day of the current week.
Answer the following statement true (T) or false (F)