What does the following code do? Assume this method is invoked by using Mystery ( 70, 80 ).
double dblY;
intX = intNumber1 + intNumber2;
dblY = intX / 2;
if ( dblY <= 60 )
{
lblResult.Text = "<= 60 ";
}
else
{
lblResult.Text = " Result is " + dblY;
}
} // end method Mystery
This code calculates the average of two numbers. The user is informed of the aver-age, but only if the average is above 60. In this case the two numbers entered are 70 and 80, which results in an average of 75, which will be displayed. The complete code reads:
// Average.cs
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace Average
{
///
/// Summary description for FrmAverage.
///
public class FrmAverage : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblResult;
private System.Windows.Forms.Label lblNumber2Out;
private System.Windows.Forms.Label lblNumber1Out;
private System.Windows.Forms.Label lblResultText;
private System.Windows.Forms.Label lblNumber2;
private System.Windows.Forms.Label lblNumber1;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmAverage()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmAverage() );
}
// calls the Mystery method to perform a calculation
private void FrmAverage_Load(
object sender, System.EventArgs e )
{
Mystery( 70, 80 );
} // end method FrmAverage_Load
// tests whether the average of the inputs is <= 60
void Mystery( int intNumber1, int intNumber2 )
{
int intX;
double dblY;
intX = intNumber1 + intNumber2;
dblY = intX / 2;
if ( dblY <= 60 )
{
lblResult.Text = "<= 60 ";
}
else
{
lblResult.Text = " Result is " + dblY;
}
} // end method Mystery
} // end class FrmAverage
}
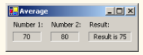
You might also like to view...
What is the efficiency of linear search?
a. O(1). b. O(log n). c. O(n). d. O(n2).
Which flowchart symbol can have more than one exit?
a. Off page connector b. Predetermined process. c. On page connector. d. Decision symbol. e. None of the above.
A program in which the user makes direct requests is a(n) ____________________ program.
Fill in the blank(s) with the appropriate word(s).
The _________ is the specific address where the web page is located
Fill in the blank(s) with the appropriate word(s).