This code should allow a user to pick a font from a Font and set the text in txtDis- play to that font. Find the error(s) in the following code, assuming that a TextBox named txtDisplay exists on a Form, along with a MenuItem named mnuitmFont.
private void mnuitmFont_Click(
object sender, System.EventArgs e )
{
FontDialog dlgFontDialog;
dlgFontDialog = new FontDialog();
dlgFontDialog.ShowDialog();
txtDisplay.Font = dlgFontDialog.Font;
} // end method mnuitmFont_Click
The code should check whether the use clicked the Cancel Button in the dialog. If
the user clicked Cancel, the method should be terminated. If the user did not click Cancel, the text should be set to the selected style. The complete incorrect code reads:
// FontChange.cs (Incorrect)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace FontChange
{
///
/// Summary description for FrmFontChange.
///
public class FrmFontChange : System.Windows.Forms.Form
{
private System.Windows.Forms.TextBox txtDisplay;
private System.Windows.Forms.Label lblSample;
private System.Windows.Forms.MainMenu mnuMain;
private System.Windows.Forms.MenuItem menuItem1;
private System.Windows.Forms.MenuItem mnuitmFont;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmFontChange()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmFontChange() );
}
// changes the font of the Form's TextBox
private void mnuitmFont_Click(
object sender, System.EventArgs e )
{
FontDialog dlgFontDialog;
dlgFontDialog = new FontDialog();
dlgFontDialog.ShowDialog();
txtDisplay.Font = dlgFontDialog.Font;
} // end method mnuitmFont_Click
} // end class FrmFontChange
}
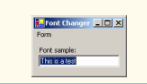
The complete corrected code should read:
// FontChange.cs (Correct)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace FontChange
{
///
/// Summary description for FrmFontChange.
///
public class FrmFontChange : System.Windows.Forms.Form
{
private System.Windows.Forms.TextBox txtDisplay;
private System.Windows.Forms.Label lblSample;
private System.Windows.Forms.MainMenu mnuMain;
private System.Windows.Forms.MenuItem menuItem1;
private System.Windows.Forms.MenuItem mnuitmFont;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmFontChange()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmFontChange() );
}
// changes the font of the Form's TextBox
private void mnuitmFont_Click(
object sender, System.EventArgs e )
{
DialogResult result;
FontDialog dlgFontDialog;
dlgFontDialog = new FontDialog();
result = dlgFontDialog.ShowDialog();
if ( result == DialogResult.Cancel )
{
return;
}
else
{
txtDisplay.Font = dlgFontDialog.Font;
}
} // end method mnuitmFont_Click
} // end class FrmFontChange
}
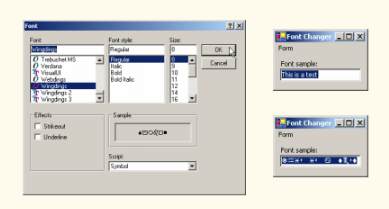
You might also like to view...
Storage is the area of a computer that temporarily holds data waiting to be processed, saved, or output.
Answer the following statement true (T) or false (F)
The ________ data type is used for fields that will be used in mathematical calculations except those involving money
Fill in the blank(s) with correct word
3-D references must be updated manually when you adjust the reference point
Indicate whether the statement is true or false
An Actions Pane Control must be displayed at runtime by a programmatic request to open it.
Answer the following statement true (T) or false (F)