Design a digital filter approximation to each of these ideal analog filters by sampling a truncated version of the impulse response and using the specified window. In each case choose a sampling frequency which is 10 times the highest frequency passed by the analog filter. Choose the delays and truncation times such that no more than 1% of the signal energy of the impulse response is truncated. Graphically compare the magnitude frequency responses of the digital and ideal analog filters using a dB magnitude scale versus linear frequency.
What will be an ideal response?
fc = 1 ; type = 'LP' ; fs = 10*fc ;
%
% Lowpass, Rectangular Window
%
hd = FIRDF(type,fc,fs,'RE',0.01) ;
N = length(h) ; Ts = 1/fs ; n = [0:N-1]' ;
F = [0:0.001:1/2]' ; [Hd,F] = DTFT(n,hd,F) ;
subplot(2,2,1) ;
p = xyplot(F*fs,20*log10(abs(Hd)),[0,fs/2,-120,0],'\itf ',...
'|H_d(\ite^{{\itj}2{\pi}{\itf}{\itT}_s} )|',...
'Times',18,'Times',14,...
'Lowpass - Rectangular Window','Times',24,'n','c') ;
subplot(2,2,2) ;
p = xyplot(F*fs,20*log10(abs(Hd)),[0,fs/10,-5,0],'\itf ',...
'|H_d(\ite^{{\itj}2{\pi}{\itf}{\itT}_s} )|',...
'Times',18,'Times',14,...
'Lowpass - Rectangular Window','Times',24,'n','c') ;
%
% Lowpass, von Hann Window
%
hd = FIRDF(type,fc,fs,'VH',0.01) ;
N = length(h) ; Ts = 1/fs ; n = [0:N-1]' ;
F = [0:0.001:1/2]' ; [Hd,F] = DTFT(n,hd,F) ;
subplot(2,2,3) ;
p = xyplot(F*fs,20*log10(abs(Hd)),[0,fs/2,-120,20],'\itf ',...
'|H_d(\ite^{{\itj}2{\pi}{\itf}{\itT}_s} )|',...
'Times',18,'Times',14,...
'Lowpass - Von Hann Window','Times',24,'n','c') ;
subplot(2,2,4) ;
p = xyplot(F*fs,20*log10(abs(Hd)),[0,fs/10,-5,0],'\itf ',...
'|H_d(\ite^{{\itj}2{\pi}{\itf}{\itT}_s} )|',...
'Times',18,'Times',14,...
'Lowpass - Von Hann Window','Times',24,'n','c') ;
% Function to design a digital filter using truncation of the
% impulse response to approximate the ideal impulse response. The
% user specifies the type of ideal filter,
%
% LP - lowpass
% BP - bandpass
%
% the cutoff frequency(s) fcs (a scalar for LP and HP a 2-vector
% for BP and BS), the sampling rate, fs, the type of window,
%
% RE - rectangular
% VH - von Hann
% BA - Bartlett
% HA - Hamming
% BL - Blackman
%
% and the allowable truncation error,
% err, as a fraction of the total impulse response signal energy.
%
% The function returns the filter coefficients as a vector.
%
% function hd = FIRDF(type,fcs,fs,window,err)
%
function hd = FIRDF(type,fcs,fs,window,err)
if fs == 0 | fs == inf | fs == -inf,
disp('Sampling rate is unusable') ;
else
fs
Ts = 1/fs ;
type = upper(type) ; window = upper(window) ;
switch type
case 'LP',
fc = fcs(1) ; zc1 = 1/(2*fc) ;
N = 200*zc1/Ts ; n = [0:N]' ;
Etotal = sum(sinc(2*fc*n*Ts).^2)
E = 0 ; N = 1 ;
while abs(E-Etotal) > Etotal*err,
N = 2*N ;
n = [0:N]' ;
E = sum(sinc(2*fc*n*Ts).^2) ;
end
delN = floor(N/10) ;
while abs(E-Etotal)1,
N = N-delN ;
n = [0:N]' ;
E = sum(sinc(2*fc*n*Ts).^2) ;
delN = floor(delN/2) ;
end
N = ceil(N/2)*2 ; % Make number of pts even
nmid = (N/2-1) ;
w = makeWindow(window,n,N) ;
hd = w.*sinc(2*fc*(n-nmid)*Ts) ;
hd = hd/sum(hd) ;
case 'BP'
fl = fcs(1) ; fh = fcs(2) ; fmid = (fh + fl)/2 ;
df = abs(fh-fl) ; zc1 = 1/df ;
N = 200*zc1/Ts ; n = [0:N]' ;
Etotal = sum((2*df*sinc(df*n*Ts).*...
cos(2*pi*fmid*n*Ts)).^2) ;
E = 0 ; N = 1 ;
while abs(E-Etotal) > Etotal*err,
N = 2*N ;
n = [0:N]' ;
E = sum((2*df*sinc(df*n*Ts).*...
cos(2*pi*fmid*n*Ts)).^2) ;
end
delN = floor(N/10) ;
while abs(E-Etotal)1,
N = N-delN ;
n = [0:N]' ;
E = sum((2*df*sinc(df*n*Ts).*...
cos(2*pi*fmid*n*Ts)).^2) ;
delN = floor(delN/2) ;
end
N = ceil(N/2)*2 ; % Make number of pts even
nmid = (N/2-1) ; n = [0:N-1]' ;
w = makeWindow(window,n,N) ;
hd = w.*2.*df.*sinc(df*(n-nmid)*Ts).*...
cos(2*pi*fmid*(n-nmid)*Ts) ;
hd = hd/sum(hd.*cos(2*pi*fmid*(n-nmid)*Ts)) ;
end
end
function w = makeWindow(window,n,N)
switch window
case 'RE'
w = ones(N,1) ;
case 'VH'
w = (1-cos(2*pi*n/(N-1)))/2 ;
case 'BA'
w = 2*n/(N-1).*(0<=n & n<=(N-1)/2) + ...
(2-2*n/(N-1)).*((N-1)/2<=n & n
case 'HA'
w = 0.54 - 0.46*cos(2*pi*n/(N-1)) ;
case 'BL'
w = 0.42 - 0.5*cos(2*pi*n/(N-1)) + ...
0.08*cos(4*pi*n/(N-1)) ;
otherwise
w = ones(N,1) ;
end
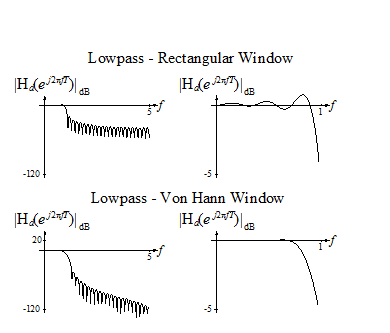
Trades & Technology
You might also like to view...
An ____ or expansion joint is used to separate a slab from an adjacent slab, wall, or column, or some other part of the structure.
A. elimination B. interference C. isolation D. escape
Trades & Technology
The area where all living and nonliving features depend on each other is called the _____
A) farm B) ecosystem C) canopy D) sublayer
Trades & Technology
A vaccine is a weakened or killed pathogen developed to evoke a strong immune response
Indicate whether the statement is true or false
Trades & Technology
When tungsten touches the molten weld pool, ____________________ pulls the contamination up onto the hot tungsten.
Fill in the blank(s) with the appropriate word(s).
Trades & Technology