What is the result of the following code?
ArrayList intList = new ArrayList();
string strOutput = "";
intList.Add( 1 );
intList.Add( 3 );
intList.Add( 5 );
foreach ( int intListItems in intList )
{
strOutput += ( " " + intListItems.ToString() );
}
MessageBox.Show( strOutput, "Mystery",
MessageBoxButtons.OK, MessageBoxIcon.Information );
This code creates an ArrayList and adds to it the values 1, 3 and 5. The values are
then appended to each other (separated by spaces) using a foreach statement, and the result is displayed in a MessageBox. The complete code reads:
// MessageBox.cs
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace MessageBoxes
{
///
/// Summary description for FrmMessageBox.
///
public class FrmMessageBox : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblOutputOut;
private System.Windows.Forms.Label lblOutput;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmMessageBox()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmMessageBox() );
}
private void FrmMessageBox_Load(
object sender, System.EventArgs e )
{
ArrayList intList = new ArrayList();
string strOutput = "";
intList.Add( 1 );
intList.Add( 3 );
intList.Add( 5 );
foreach ( int intListItems in intList )
{
strOutput += ( " " + intListItems.ToString() );
}
MessageBox.Show( strOutput, "Mystery",
MessageBoxButtons.OK, MessageBoxIcon.Information );
} // end method FrmMessageBox_Load
} // end class FrmMessageBox
}
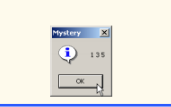
You might also like to view...
Forum rooms provide a synchronous environment in which a group of participants share text-based information.
Answer the following statement true (T) or false (F)
Find the adjacency and Laplacian spectra of the graphs shown in Figure 8.1 .
What will be an ideal response?
A(n) _________ is a private, secure path across a public network that is set up to allow authorized users private, secure access to a network. A. virtual private network (VPN) B. local private network (LPN) C. managed private network (MPN) D. web private network (WPN)
Fill in the blank(s) with the appropriate word(s).
What type of parameter requires you to indicate the parameter's type and name, and the method receives a copy of the value passed to it?
A. reference parameter B. output parameter C. input parameter D. value parameter