The following lines of code are supposed to create a DateTime variable and increment its hour value by two. Find the error(s) in the code.
DateTime dtmNow = DateTime.Now;
dtmNow.AddHours( 2 );
Method AddHours does not actually increment the DateTime variable, but instead
returns a new DateTime variable with the updated value. Thus, the preceding code will not successfully add two hours to dtmNow. The complete incorrect code reads:
// ChangeTime.cs (Incorrect)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace ChangeTime
{
///
/// Summary description for FrmChangeTime.
///
public class FrmChangeTime : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblTwoHours;
private System.Windows.Forms.Label lblCurrentTime;
private System.Windows.Forms.Label lblTwoHoursText;
private System.Windows.Forms.Label lblCurrentText;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmChangeTime()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmChangeTime() );
}
// adds two hours to the current time and shows the result
private void FrmChangeTime_Load(
object sender, System.EventArgs e )
{
DateTime dtmNow = DateTime.Now;
dtmNow.AddHours( 2 );
lblCurrentTime.Text = Convert.ToString( DateTime.Now );
lblTwoHours.Text = Convert.ToString( dtmNow );
} // end method FrmChangeTime_Load
} // end class FrmChangeTime
}
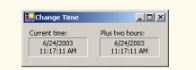
The complete correct code should read:
// ChangeTime.cs (Correct)
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
namespace ChangeTime
{
///
/// Summary description for FrmChangeTime.
///
public class FrmChangeTime : System.Windows.Forms.Form
{
private System.Windows.Forms.Label lblTwoHours;
private System.Windows.Forms.Label lblCurrentTime;
private System.Windows.Forms.Label lblTwoHoursText;
private System.Windows.Forms.Label lblCurrentText;
///
/// Required designer variable.
///
private System.ComponentModel.Container components = null;
public FrmChangeTime()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent
// call
//
}
///
/// Clean up any resources being used.
///
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
// Windows Form Designer generated code
///
/// The main entry point for the application.
///
[STAThread]
static void Main()
{
Application.Run( new FrmChangeTime() );
}
// adds two hours to the current time and shows the result
private void FrmChangeTime_Load(
object sender, System.EventArgs e)
{
DateTime dtmNow = DateTime.Now;
lblCurrentTime.Text = Convert.ToString( DateTime.Now );
lblTwoHours.Text = Convert.ToString( dtmNow );
} // end method FrmChangeTime_Load
} // end class FrmChangeTime
}
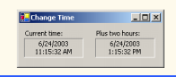
You might also like to view...
An SQL statement that defines the criteria that should be applied when a query is run
a. WHERE clause b. WHEN clause c. WHAT clause
Which of the following best describes a system-specific policy
A. Used to create a management-sponsored computer security program B. Focuses on policy issues that management has decided for a specific system C. Establishes the overall approach to computer security D. Addresses specific issues of concerns to the organization
Menus are customized to __________. (Choose all that apply.)
a. provide shortcuts to frequently used applications b. remove applications that are infrequently used c. change fonts, color schemes, and window behaviors d. group applications by use
In ingress filtering, the firewall examines packets________
A. exiting the network from the outside. B. entering the network from the inside. C. entering the network from the outside. D. exiting the network from the inside.